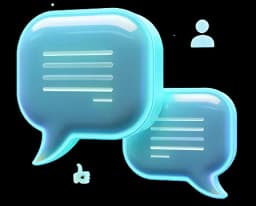
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Complete source code available till this point of lesson is available at
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:02 So let's implement Auth.
00:00:04 In this course, I'll teach you how to implement Auth completely from scratch using the latest version of NextAuth, recently renamed to AuthJS,
00:00:15 the authentication library you want to use for the web, completely free and open source.
00:00:20 So click Get Started and head over to Installation.
00:00:24 We'll be using Next.js with npm.
00:00:27 So let's copy the installation command, open up a new terminal window, and run npm install next-auth at beta.
00:00:36 After that, we need to set up our environment by declaring our auth secret.
00:00:41 So let's run mpx auth secret.
00:00:44 It's going to ask us whether we want to install the package.
00:00:47 I'll say yes.
00:00:48 And it's going to create a new .env.local with our auth secret.
00:00:54 You can find it right here.
00:00:56 Next, we need to configure it by creating the Auth.js config file and object.
00:01:01 So we have some specific information for Next.js.
00:01:04 Start by creating a new Auth.ts file in the root of your application.
00:01:10 So that's going to be right here outside of everything, auth.ts.
00:01:14 In there, we can copy the code that they give us, import nextAuth from nextAuth, where we export handlers, sign in and sign out functions,
00:01:22 as well as auth, which will give us the information about the current session.
00:01:26 Next, we have to add the route handlers by creating a new folder under app, API, auth, and then square brackets dot dot dot nextAuth route.ts.
00:01:36 Let's first create it.
00:01:37 And then I'll explain why are we using this specific syntax.
00:01:41 So we can go over to the app, create a new folder called API, create another folder called auth within it, then create a new folder,
00:01:53 square brackets dot dot dot next auth.
00:01:57 like this, and then within it, create a new route.ts.
00:02:02 Now, why are we using this file naming structure with square brackets dot dot dot something?
00:02:07 Well, as you might know, square brackets denote a dynamic route segment.
00:02:13 and dot dot dot represents a catch-all route, which means it will match any number of path segments after it.
00:02:20 That's explained a bit more under the catch-all segments of dynamic routing.
00:02:24 For example, if you have the app shop slug page, it'll match both shop clothes, as well as shop clothes tops, as well as shop clothes tops t-shirts,
00:02:36 and so on.
00:02:37 In this case, it helps the Auth.js package to route things properly.
00:02:41 It makes sure that we handle the sign-in, sign-out routes, or any other authentication routes.
00:02:47 Now, what do we want to put in this route.ts?
00:02:50 Well, we can follow what the docs say.
00:02:52 We need to import the handlers, and then we have to export get and post route handlers.
00:02:57 Finally, we need to create one last file called middleware.ts that's explained here, where we can copy and paste the code they provide,
00:03:06 export auth as middleware from next auth.
00:03:10 Now, the basis for our auth has been set up, but we have to set up different authentication methods, such as the providers,
00:03:17 GitHub and Google.
00:03:19 Let me show you how you can do that.
00:03:20 Head over to github.com, click your profile icon, and then go to settings.
00:03:25 Scroll to the bottom where you can see developer settings, and then don't create a new GitHub app, but create a new OAuth app.
00:03:34 You can see I have a couple.
00:03:36 You can click new OAuth app and give it a name.
00:03:41 I'll use Devflow, and I'll set up the homepage URL.
00:03:44 In this case, it has to be HTTP, not HTTPS, colon, forward slash, forward slash, localhost, 3000. And we also need to set up the authorization callback,
00:03:57 which is gonna start with the same URL, but it's gonna point to the route we just created, forward slash API, forward slash auth,
00:04:05 And in this case, we don't really need to enable device flow as it's used to authorize users for headless applications.
00:04:17 It's designed for devices that don't have an easy way to input text like smart TVs or IoT devices or gaming consoles.
00:04:24 So by enabling this, a user is prompted to scan the QR code and then log in with their different device.
00:04:30 You don't really have to enable it, but if you want somebody to view your app on a smart TV, then feel free to turn it on.
00:04:36 Now, register your application, and you'll be given your client ID.
00:04:40 So, let's copy it, go back to our code, to the .env.local, and let's paste it right here.
00:04:46 This will be our auth underscore github underscore id.
00:04:51 So you can say equal to, and then we have our string.
00:04:54 After that, you can also generate a new client secret.
00:04:57 You'll have to authenticate your account to do that, and then you can just copy it.
00:05:01 Back in your code, you can say auth underscore github underscore secret, and make it equal to the secret that you just copied.
00:05:10 And you can see that now we have the general auth secret, we have the auth github ID, and the auth github secret.
00:05:16 Now you don't have to wrap these in string signs, but I usually like to do it just to get syntax highlighting and to make sure that everything in here
00:05:25 actually is a string.
00:05:26 Now we want to head back over to auth.ts and we can add our first provider.
00:05:33 GitHub.
00:05:34 Coming from NextAuthProviders GitHub.
00:05:37 And now that we have the general setup done and ready to go, in the next lesson, I'll teach you how to fully implement GitHub OAuth so you can sign your
00:05:46 users into your application.