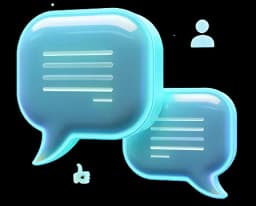
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Similar to how you used the special convention file loading.js in the previous lesson to see loaders, you can use error.js in exactly the same way.
When you define an error.js file in a route segment, Next.js automatically wraps that segment and its nested children in a React Error Boundary (Similar to React Suspense Boundary for loading states). This means that if an error occurs within that segment, it will be caught, and a fallback UI (the component exported from error.js) will be displayed instead of the default error page.
The only difference is this error.js file has to be a client component. Why?
Because the error.js file is meant to catch errors that happen while your app is rendering, and Error Boundaries handle issues during rendering, lifecycle methods, and constructors of child components—they need to be client components to work properly.
By making the error.js file a client component, Next.js can properly handle errors within your application by using React's Error Boundaries mechanism.
Error Boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed.
Suspense is a React feature that allows components to "wait" for something before rendering, such as data fetching or code splitting. Suspense Boundaries enable you to specify loading states for components that are waiting for asynchronous operations to complete
To see error.js in action, go to app/(root)/community/page.tsx route and create a new file error.js inside it.
In that error.js file, add this code that shows there is an error and allows you to retry it,
'use client'
import { useEffect } from 'react'
export default function Error({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
useEffect(() => {
// Log the error to an error reporting service
console.error(error)
}, [error])
return (
<div>
<h2>Something went wrong!</h2>
<button
onClick={
// Attempt to recover by trying to re-render the segment
() => reset()
}
>
Try again
</button>
</div>
)
}
To see if this file even works or not, let’s deliberately throw an error in the community page. Go to app/(root)/community/page.tsx and purposely throw an error somewhere like this,
const Page = async ({ searchParams }: RouteParams) => {
const { page, pageSize, query, filter } = await searchParams;
throw new Error("Error");
const { success, data, error } = await getUsers({
page: Number(page) || 1,
pageSize: Number(pageSize) || 10,
query,
filter,
});
const { users, isNext } = data || {};
return (
<>
</>
);
};
export default Page;
Now, head over to http://localhost:3000/community, and you should see the content from error.js. It should be working fine.
Feel free to experiment with it, customize the error.js content to make your error page look even cooler, and use it for all pages if necessary.
It’s on you 😉
Complete source code for this lesson is available at
00:00:00 Let me also show you how to implement page-level error handling in Next.js.
00:00:05 It's just about creating a single error file and adding some content to it.
00:00:10 So if we're going to continue with the community page, you can add it right here in the community folder and create a new file called error.tsx.
00:00:19 We can quickly read more about it on Next.js documentation, where you can see that you can create a new error file, so error.js file,
00:00:28 and it looks something like this.
00:00:30 They give an example for an app dashboard, app-dashboard-error-tsx.
00:00:34 So you can actually copy this.
00:00:36 Just Google Next.js error handling and scroll down to this part, and copy and paste it right here.
00:00:42 We start by defining this file as useClient because error boundaries must be client components.
00:00:49 Then we import the useEffect.
00:00:51 We destructure the error and the reset from the params of this function.
00:00:57 We call the useEffect where we can lock the error or send it to some kind of an error logging system.
00:01:02 And we tell the user that something went wrong and we can give them a try again button to try to reset it.
00:01:07 So now let me purposely throw an error in the community page.
00:01:11 I'll head over to page community and I'll throw a new error right here.
00:01:16 Throw new error.
00:01:18 I'll say this is a test error.
00:01:21 If I save it and reload, you can see something went wrong.
00:01:24 Try again.
00:01:25 So technically we have caught this error right here by creating this simple file.
00:01:30 Pretty cool, right?
00:01:31 But keep in mind, this is a page-based error.
00:01:34 So we technically cannot see anything else on the page with this type of approach.
00:01:38 So what we have done before in the application is even better, where if something breaks, we will hide or error out only that part of the code while the
00:01:48 rest will still be visible, allowing you to use the rest of the app's functionalities.