Lifetime access to this course
Implement Answer Delete Functionality
You’re almost there. Just like you did with deleting questions, it’s time to implement the delete functionality for answers. Yes — it’s a bit of repetition, but you know the drill by now. Start with the usual setup (you probably don’t even need to pause to think about it at this point) - Define type for params
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
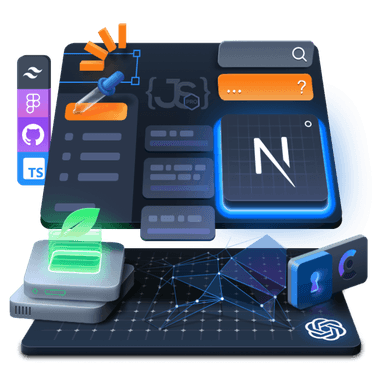
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
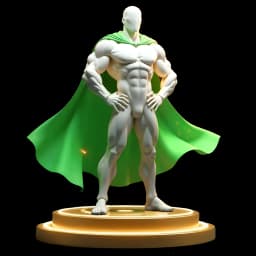
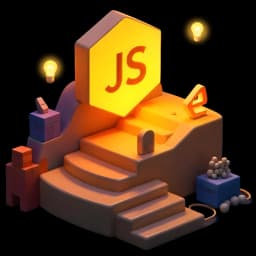
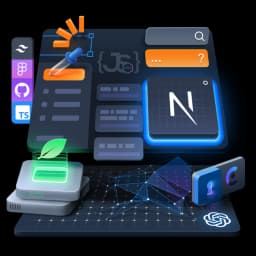
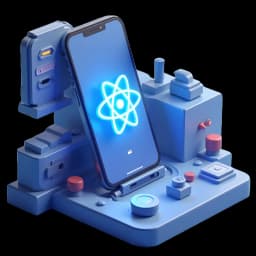
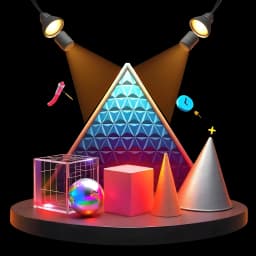
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access