Lifetime access to this course
Implement Question Delete Functionality
It’s time to implement a feature that allows users to delete the questions they've created. So, how do you approach it? Based on everything you've learned so far, the initial steps should feel familiar and pretty straightforward. Here's what you'll do: - Create a server action function
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
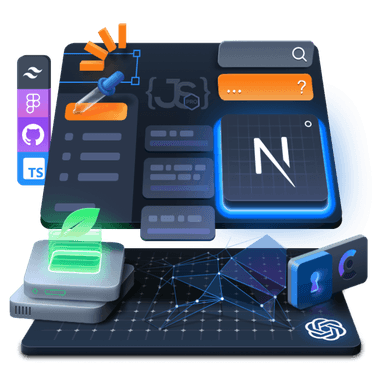
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
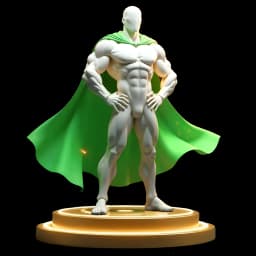
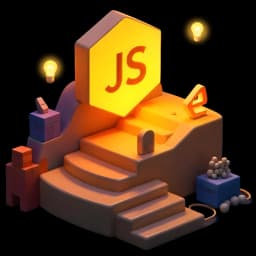
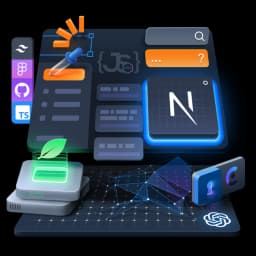
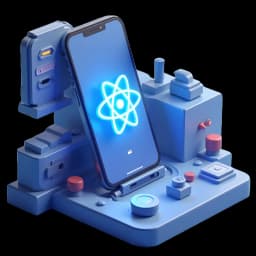
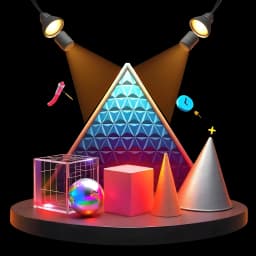
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access