Lifetime access to this course
How to SEO-optimize websites in Nextjs?
You might be wondering, "Aren’t we making two requests on the same page? Doesn’t that hurt performance?" The good news is—not at all if you’re using fetch requests 😄 Next.js automatically memoizes (caches) fetch calls across layouts, pages, and server components when they request the same data. So even if you call the same function in both generateMetadata() and your page component, it won’t run twice. However, if you're using server actions, you'll need to handle memoization yourself.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
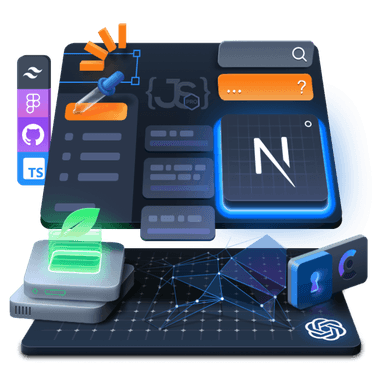
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
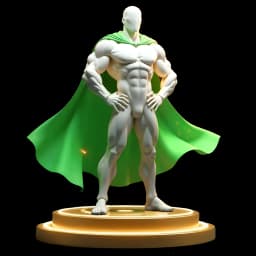
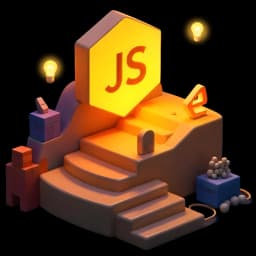
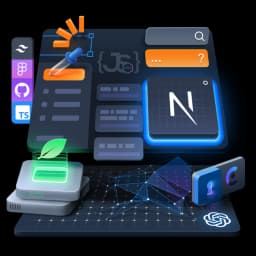
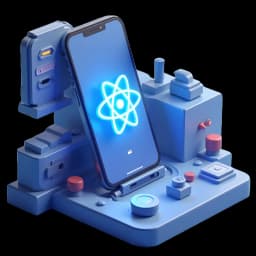
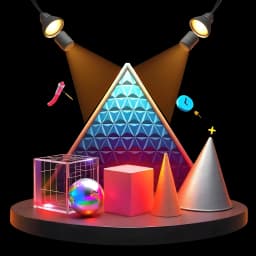
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access