Lifetime access to this course
Build interactions and reputation
If you’ve been paying attention to the architecture so far, you might have noticed that the User model already has a field for reputation. But we’re not showing it in the UI yet, are we? Let’s start with that. Open app/(root)/profile/[id]/page.tsx and pass the reputation points to the Stats component. You can show it wherever you like, but since reputation is tied to badges and similar info, it makes sense to place it nearby. I will keep it there. ```js /app/(root)/profile/[id]/page.tsx;
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
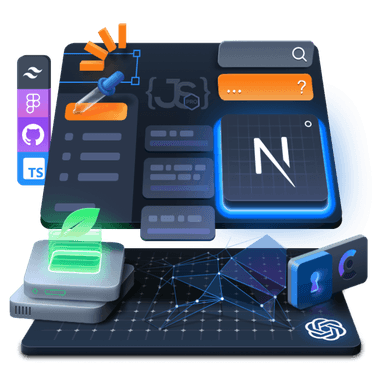
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
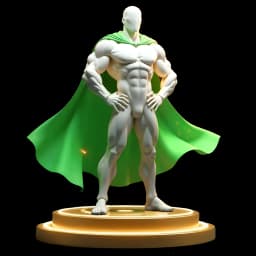
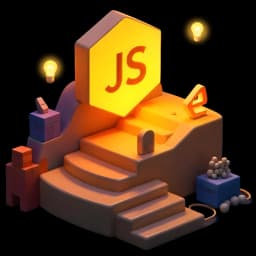
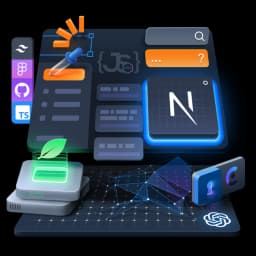
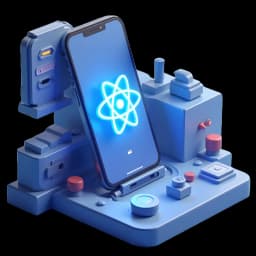
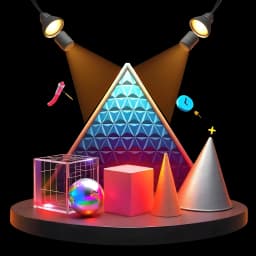
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access