Lifetime access to this course
Build Recommendation System
You’ve made it to the final step of building this feature. Recommendation. Now, pause for a second—what pops into your head when you hear "recommendation"? After everything you’ve built so far, does it feel like there’s going to be some complex magic involved? If you’re thinking it’s some kind of mysterious data science trick—nah, not really. You’re overthinking it.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
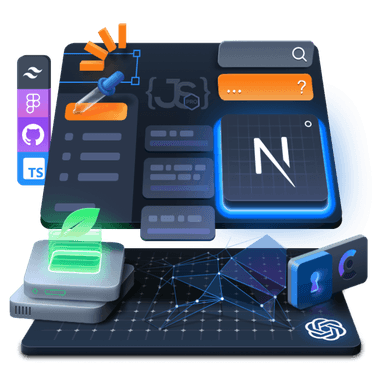
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
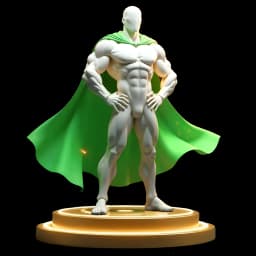
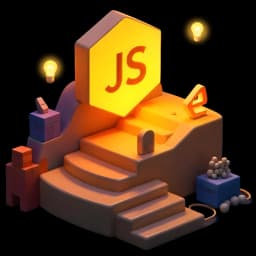
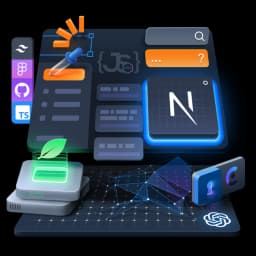
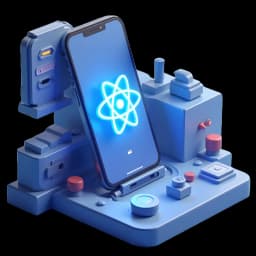
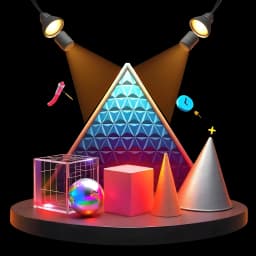
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access