Lifetime access to this course
Call Interactions on Actions
There’s a popular saying in the dev world—solve the problem first, then write the code. And that’s exactly what you did in the last lesson by breaking down the logic before jumping into implementation. Now, it’s time to put that system logic to work and see the results in action. So… what’s next Ask yourself—Where should we trigger this createInteraction function? For what kind of actions, and how? Simple.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
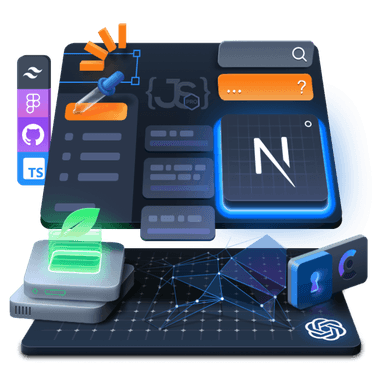
The Ultimate Next.js 15 Course
$599
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
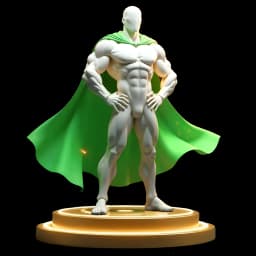
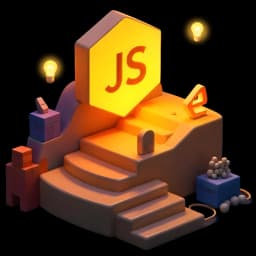
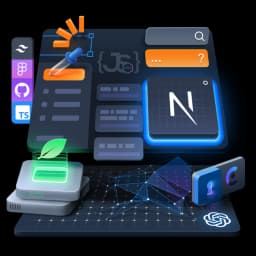
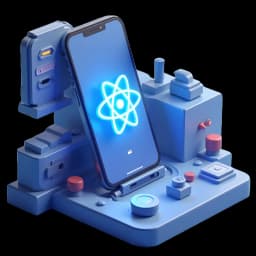
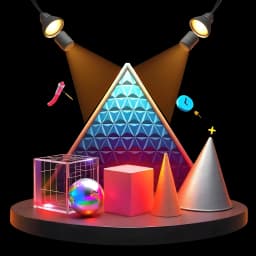
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access