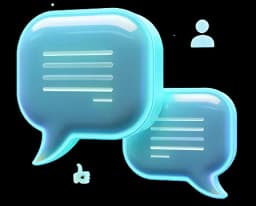
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Welcome Back! In the previous lessons, we explored sliders, color pickers, checkboxes, and folders in
Now, let’s move on to another essential GUI feature—Dropdown (Select) Controls! ⬇️🎛️ Dropdowns allow users to select a value from a predefined list. This is useful for:
A dropdown (select) control allows users to choose from a list of predefined options.
In , we use:
gui.add(object, "property", [option1, option2, option3]);
Parameters:
Let’s create a dropdown to switch between different geometries (cube, sphere, cylinder).
const shapeOptions = { Cube: "cube", Sphere: "sphere", Cylinder: "cylinder" };
const settings = { shape: "cube" };
gui.add(settings, "shape", shapeOptions).onChange((value) => {
scene.remove(cube); // Remove previous shape
let geometry;
if (value === "cube") geometry = new THREE.BoxGeometry();
if (value === "sphere") geometry = new THREE.SphereGeometry(0.7, 32, 32);
if (value === "cylinder") geometry = new THREE.CylinderGeometry(0.5, 0.5, 1, 32);
cube = new THREE.Mesh(geometry, material);
scene.add(cube);
});
✅ Now, selecting an option from the dropdown menu will replace the existing object with a new shape dynamically! 🔄
Let’s allow users to select different animation types.
const animationOptions = {
None: "none",
Rotate: "rotate",
Bounce: "bounce",
};
```js
#### Step 2: Store the Selected Animation
```js
const settings = { animation: "none" };
gui.add(settings, "animation", animationOptions);
function animate() {
requestAnimationFrame(animate);
if (settings.animation === "rotate") {
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
}
if (settings.animation === "bounce") {
cube.position.y = Math.sin(Date.now() * 0.002) * 2;
}
renderer.render(scene, camera);
}
animate();
✅ Now, selecting an animation type will apply a different movement style to the object! 🔄🔁
To keep the GUI panel clean, we can group dropdown menus inside a folder.
const dropdownFolder = gui.addFolder("Dropdown Controls");
dropdownFolder.add(settings, "shape", shapeOptions).onChange((value) => {
scene.remove(cube);
let geometry;
if (value === "cube") geometry = new THREE.BoxGeometry();
if (value === "sphere") geometry = new THREE.SphereGeometry(0.7, 32, 32);
if (value === "cylinder") geometry = new THREE.CylinderGeometry(0.5, 0.5, 1, 32);
cube = new THREE.Mesh(geometry, material);
scene.add(cube);
});
dropdownFolder.add(settings, "material", materialOptions).onChange((value) => {
let newMaterial;
if (value === "basic") newMaterial = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
if (value === "lambert") newMaterial = new THREE.MeshLambertMaterial({ color: 0x00ff00 });
if (value === "phong") newMaterial = new THREE.MeshPhongMaterial({ color: 0x00ff00 });
cube.material = newMaterial;
});
dropdownFolder.add(settings, "animation", animationOptions);
✅ Now, the Dropdown Controls folder will contain Shape, Material, and Animation selections! 📂🔽
In the next lesson, we’ll explore Buttons in , allowing users to trigger actions like resetting values or generating random colors! 🔘🚀
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.