Lifetime access to this course
Folders
In the previous lessons, we explored sliders (range controls), color pickers, and checkboxes in lil-gui Now, let’s move on to another essential feature—Folders! 📂✨ Using folders, we can organize multiple GUI controls into collapsible sections, making the interface cleaner and easier to navigate. By the end of this lesson, you’ll be able to: Group related controls together in folders. Keep the GUI organized and clutter-free. Use nested folders for better UI structure.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
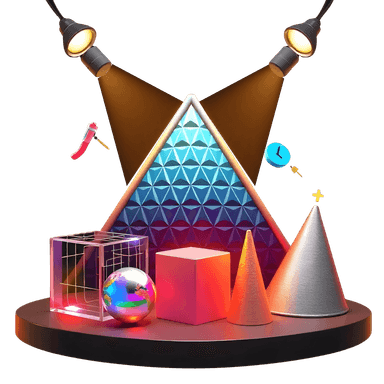
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
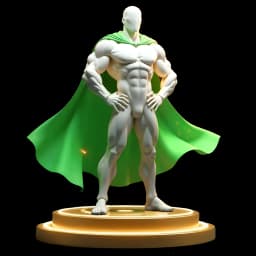
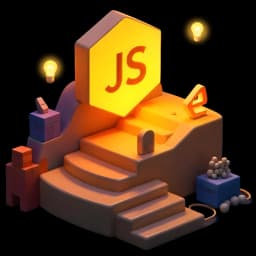
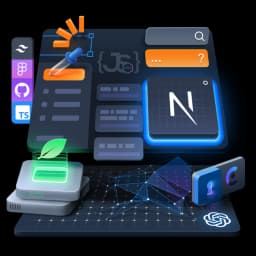
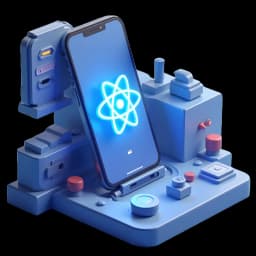
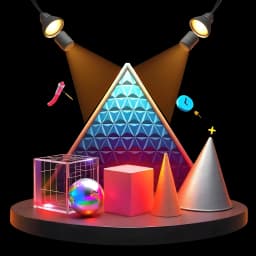
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access