Lifetime access to this course
Range (Slider)
In the previous lesson, we explored why lil-gui is a powerful GUI library for Three.js . Now, we’ll dive into one of the most commonly used controls in lil-gui —the Range (Slider). Sliders are essential for dynamically adjusting values like: Object transformations (size, position, rotation). Material properties (opacity, roughness, metalness). Light intensity (brightness, distance, color blending). By the end of this lesson, you’ll know how to add sliders to your GUI panel and make real-time changes to your 3D objects.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
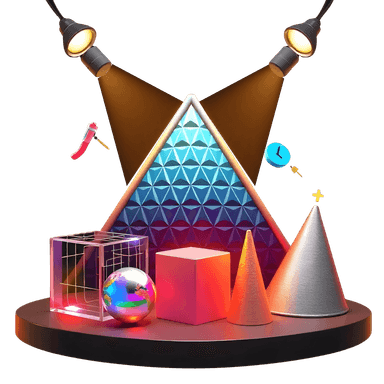
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
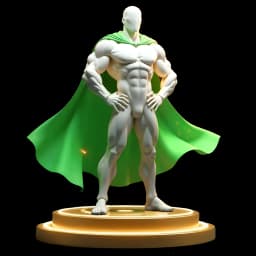
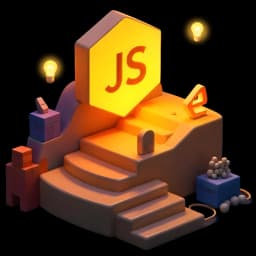
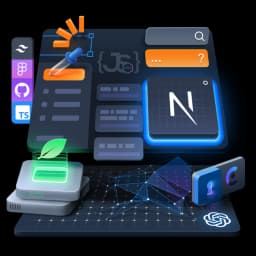
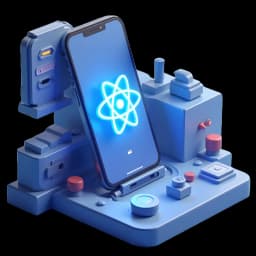
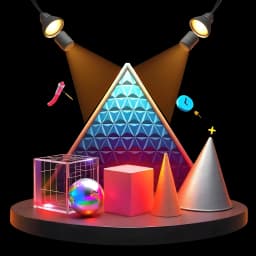
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access