Lifetime access to this course
Basic Three.js Structure
Creating Your First 3D Scene in Three.js Welcome Back! Hey everyone! In this lesson, we’re going to create our first 3D scene together. By the end of this lesson, you’ll have a pink background with a spinning red cube . Sounds fun, right? Let’s jump in! ### What Are We Building? We’re going to create a simple 3D scene with a rotating cube. This might sound fancy, but trust me, it’s not as complicated as it seems. We’ll break it down step by step.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
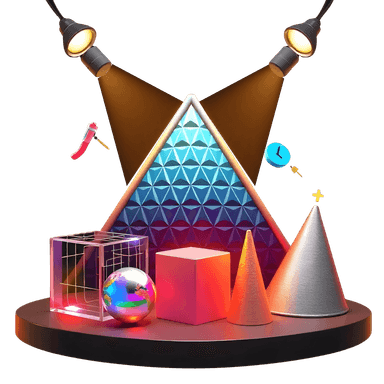
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
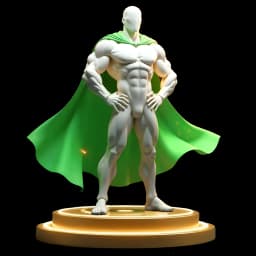
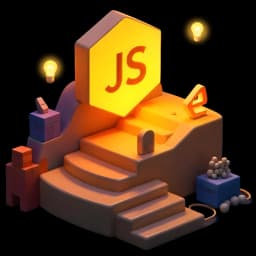
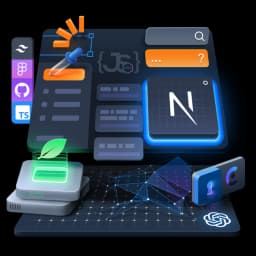
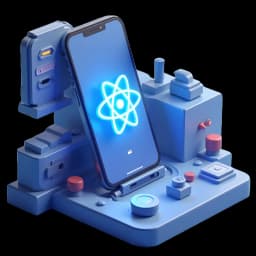
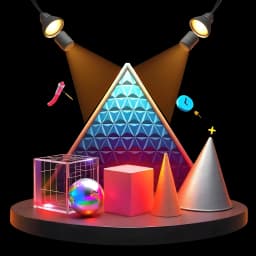
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access