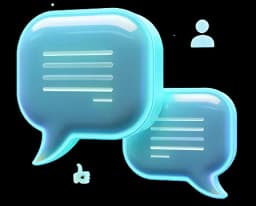
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Alright — Now that we know what Three.js is and why it’s so awesome, it’s time to actually start building something! 🛠️✨
But before we jump into magical 3D worlds, let’s take a tiny step back — and look at
If you've ever built a simple website before, you know the usual trio:
You usually have a folder with files like this:
index.html
styles.css
main.js
The browser reads these and creates your web page. Simple and powerful!
But if we want to move into ... 👉 we’ll need something extra: something that can act like a canvas for us to paint our 3D scenes onto.
Imagine a blank painting canvas. 🎨 An empty space where you can draw anything you want.
In web development, we have something exactly like that: the canvas tag!
<canvas class="world"></canvas>
This little canvas is about to become your window into another world. 🌌
At a professional level, we don’t just link Three.js from a URL.
Instead, we usually using a package manager like .
This way, our project is more organized, and we have full control over versions. 🔥
You can always find the official Three.js install guide 👉 here
To install Three.js, simply open your terminal and run:
npm install three
That’s it!
Three.js will be added inside your project's node_modules folder.
stands for Node Package Manager.
It's a tool that helps you like Three.js into your project —
without needing to manually download and add files yourself.
Imagine it like an app store, but for code packages! 📦✨
With just one command like:
npm install three
you grab the latest version of Three.js and it’s ready to use in your project!
— we'll use it step-by-step together throughout this course. 🚀
Now you have all the power of Three.js loaded into your page!
Here’s what your project files will look like:
index.html
styles.css
main.js
And your index.html will look something like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>My First Three.js Scene</title>
<script src="https://cdn.jsdelivr.net/npm/three@0.160.2/build/three.min.js"></script>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<canvas class="world"></canvas>
<script src="script.js"></script>
</body>
</html>
🚀 Let’s Make Your First 3D Cube! Now, inside your mian.js, let’s write some magic:
Now that Three.js is installed, instead of relying on a global script tag, let's properly into our JavaScript files:
// Import Three.js
import * as THREE from 'three';
This way, you can use all the Three.js features cleanly inside your code.
Your main.js would now look like this:
import * as THREE from 'three';
// Create a scene
const scene = new THREE.Scene();
// Create a camera
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
// Create a renderer
const renderer = new THREE.WebGLRenderer({ canvas: document.querySelector('canvas.world') });
renderer.setSize(window.innerWidth, window.innerHeight);
// Create a cube
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// Animate the scene
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
And just like that... ✨ You have a spinning cube in 3D! ✨
You literally just created a mini universe inside your browser! 😎🌍
Don't worry, we will learn what's happening in the next lesson.
✅ Now your project is using a real professional setup — clean, maintainable, and ready to scale bigger!
We’ll dive deeper into the of Three.js — learning how scenes, cameras, lights, and objects all work together!
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.