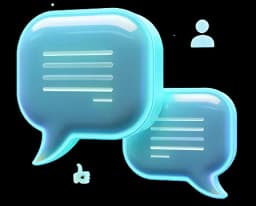
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Welcome back! 👋
Today, we’re diving into one of the most exciting building blocks in Three.js—.
Ever looked at a spinning cube, a realistic game character, or even a simple 3D logo on a website and wondered how it’s made? The answer usually starts with a mesh. And by the end of this lesson, you'll know exactly what that means—and how to create one yourself.
Grab a cup of coffee, and let’s get into it ☕✨
Think of yourself as a digital sculptor. You start with a block of clay to shape your idea—that’s the geometry. Then you paint and polish it—that’s the material.
In 3D, we do the same thing.
When you combine them, you get a Mesh—a fully formed 3D object that you can render, move, rotate, and animate.
Official DocsA geometry in Three.js is just a set of points (called vertices) in 3D space. These points are connected to form edges and faces, giving your object its form.
You don’t have to define every point manually. Three.js gives you tons of built-in shapes like:
For example, a cube has 8 vertices and 12 edges—already handled for you with:
const geometry = new THREE.BoxGeometry(1, 1, 1);
Now that you’ve got a shape, you’ll want to style it. That’s where materials come in. They define how your object looks and reacts to light.
Example:
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
This gives your mesh a flat green color with no lighting effects.
Meshes are everything in your 3D scene.
Whether it’s a simple spinning cube or a complex animated character, it all starts with a mesh.
Let’s create a basic green cube:
import * as THREE from 'three';
const scene = new THREE.Scene();
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
🎉 That’s it! You’ve just created your first mesh and added it to your scene.
Now that you understand what meshes are and how to create them, you’re ready to move on. In the next few lessons, we’ll:
Meshes are the foundation of everything you’ll build in 3D.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
00:00:00 Now that you know the basics of 3JS and 3D rendering, let's dive deeper into the foundational concept, 3D objects.
00:00:09 A 3D object is anything you can see or interact with in a 3D space.
00:00:15 There are many different types of 3D objects in 3JS, each with its own purpose.
00:00:20 A mesh is the most common type of 3D object.
00:00:23 It's made by combining geometry and a material.
00:00:27 For example, a cube in 3JS is created by combining a box geometry with a material and turning it into a mesh.
00:00:36 More on this later, as it's super important.
00:00:39 Points are another type of 3D object, and they're a collection of small dots in a 3D space, often used to create effects like stars or particles.
00:00:49 A line represents a simple connection between points in a 3D space.
00:00:55 It's often used to draw shapes, grids, or wireframes.
00:00:59 A group is a collection of multiple objects.
00:01:02 Think of it as a div in HTML, which acts as a container to organize different elements.
00:01:09 It allows us to transform many objects together.
00:01:12 Sprite, a 3D object, not a drink, is an object that always faces the camera.
00:01:18 It means it'll always be visible, no matter where we look.
00:01:22 You can use it for billboards, icons, or particles in a scene.
00:01:26 You might think that lights and cameras are also 3D objects, but they're not.
00:01:32 They're more like separate entities or tools that influence how 3D objects are seen.
00:01:38 And you know how everything in JavaScript is an object?
00:01:42 Well, that's because everything inherits some basic properties.
00:01:46 It's the same in Three.js.
00:01:49 All elements I've mentioned, like meshes, points, lines, and more, start from the base JavaScript class called Object3D and extend its properties.
00:02:00 Provides a common set of properties and methods for performing transformations, such as changing position, rotation, and scale for all types of entities
00:02:11 in the 3D scene.
00:02:12 Let me show you the most common properties.
00:02:15 Position refers to where an object is located in the 3D world.
00:02:20 It's described using three numbers, X, Y, and Z.
00:02:24 X axis moves the object left or right, Y axis moves the object up or down, and Z axis moves the object closer or farther away from you.
00:02:35 Rotation refers to how an object is turned or spun around in the 3D world.
00:02:42 You can rotate 3D objects around the X, Y and Z axis, like stretching your head.
00:02:48 X-axis tilts the object forward or backward, like nodding your head.
00:02:53 Y axis turns the object left or right, like shaking your head no, and Z axis spins the objects clockwise or counterclockwise,
00:03:02 like turning a steering wheel.
00:03:04 Scale refers to how big or small an object is in the 3D world.
00:03:09 Scaling an object along the X, Y, and Z axis can make it larger or smaller.
00:03:16 X controls how wide the object is, Y controls how tall the object is, and Z controls how deep it is.
00:03:25 You can combine position, rotation, and scale to place an object exactly where you want it in the 3D scene, orient it in the desired direction,
00:03:35 and size it appropriately.