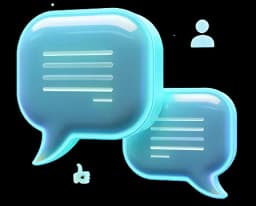
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Imagine you’re sculpting your own shapes in a vast, empty 3D space. So far, we’ve created rectangles, boxes spheres, torus, but what if you want complete control over your geometry? What if you want to build something unique—a shape that doesn’t provide out of the box?
Welcome to ! In this lesson, we’ll dive into the core of 3D modeling by manually defining a using vertices. This is where we start thinking like 3D artists and engineers.
Most built-in geometries in (like or ) are made up of vertices (points in space) and faces (triangles that connect those points).
A lets us define these elements ourselves, giving us full creative freedom.
To create a shape, we must:
Let’s break it down with a .
A is the simplest 3D shape because it has only three vertices. In , a vertex is represented as a Vector3—a point with (x, y, z) coordinates.
Let’s create three points for our :
const vertices = [
new THREE.Vector3(0, 1, 0), // Top vertex
new THREE.Vector3(-1, -1, 0), // Bottom left vertex
new THREE.Vector3(1, -1, 0) // Bottom right vertex
];
Think of it as drawing a with three dots in space.
In , we use BufferGeometry to define custom shapes. This class allows us to store vertex data efficiently.
Here’s how we set up our :
const geometry = new THREE.BufferGeometry();
Next, we need to convert our Vector3 points into a format that can understand—a simple array of numbers:
const verticesArray = new Float32Array([
0, 1, 0, // Top vertex
-1, -1, 0, // Bottom left vertex
1, -1, 0 // Bottom right vertex
]);
We then attach this data to our BufferGeometry using a position attribute:
geometry.setAttribute(
'position',
new THREE.BufferAttribute(verticesArray, 3) // 3 values per vertex (x, y, z)
);
Now, our exists as a set of points in 3D space! 🎉
Right now, our is invisible because we haven’t assigned a material yet. Since we haven’t introduced materials in this course, let’s use wireframe mode to make it visible:
const wireframeMaterial = new THREE.LineBasicMaterial({ color: 0xff0000 });
const triangleMesh = new THREE.LineLoop(geometry, wireframeMaterial);
scene.add(triangleMesh);
Here’s what’s happening:
When you run this, you’ll see a glowing red outline in your scene! 🔺
So far, we’ve created a wireframe . But what if we want to fill it so it looks solid?
Instead of LineLoop, we need to create a mesh (a filled 3D object):
const solidMaterial = new THREE.MeshBasicMaterial({ color: 0x00ff00, side: THREE.DoubleSide });
const solidTriangle = new THREE.Mesh(geometry, solidMaterial);
scene.add(solidTriangle);
Now, the is filled with green and appears solid! 🎨
Note: The side: THREE.DoubleSide property ensures the is visible from both the front and back.
Now that you’ve built a custom triangle, here are a few challenges to try:
Congratulations! 🎉 You’ve officially completed the Mesh Module in !
We started with basic geometries, like PlaneGeometry, which introduced you to how shapes are created in a 3D space. Then, we gradually moved towards more customized geometries, ultimately defining our own from scratch.
Over the past lessons, you’ve explored:
Every object you see in Three.js — no matter how complex — is built on these core principles.
And now that you understand them, you’re ready to go from shaping objects to interacting with them.
What if you could change the shape of a sphere in real time?
What if you could tweak materials, toggle wireframes, or animate a camera — all with sliders and buttons?
That’s exactly what we’ll dive into next.
The module will teach you how to use tools like to control your 3D world with ease.
Let’s bring your scene to life — not just with objects, but with real-time control.
🎛️ Let’s jump into GUI Controls!
See you in the next module! 👋✨
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.