Lifetime access to this course
Geometries
In the previous chapter, we learned that a mesh is made up of two things: a geometry (the shape) and a material (the look). Today, we're zooming in on that first piece — the one that gives our 3D object its form and structure. Geometries are the foundation of all 3D shapes in Three.js. Whether you’re building a cube, a donut, or a custom spaceship, it all starts with defining the geometry. So, let’s roll up our sleeves and explore the fascinating world of geometries in Three.js ! ---
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
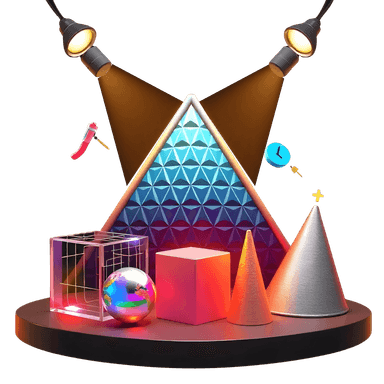
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
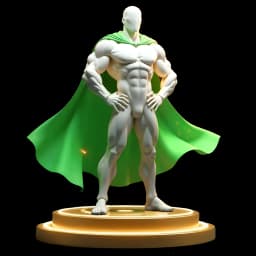
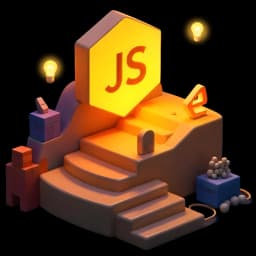
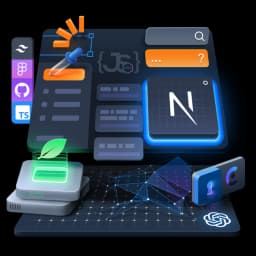
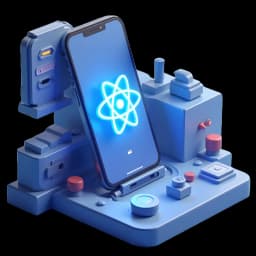
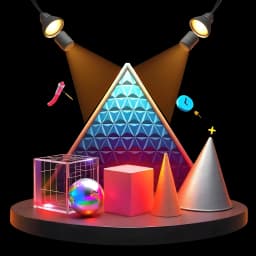
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access