Lifetime access to this course
SphereGeometry
In the previous chapter, we delved into the BoxGeometry , one of the most fundamental geometries in Three.js . Today, we’re shifting our focus to another essential geometry: the SphereGeometry . Spheres are everywhere in the 3D world—from planets and balls to bubbles and even abstract art. Understanding how to create and manipulate spheres is a crucial skill in your 3D toolkit. Sample image By the end of this chapter, you’ll know exactly what a SphereGeometry is, how to create one, and how to customize it to fit your creative vision. Let’s dive in! ### What is a SphereGeometry ?
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
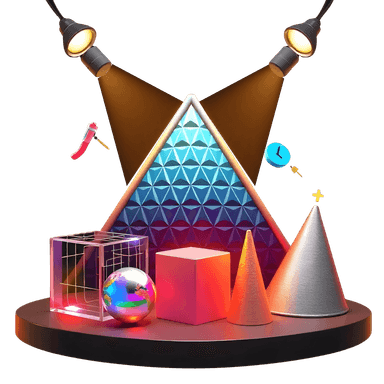
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
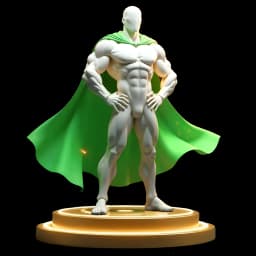
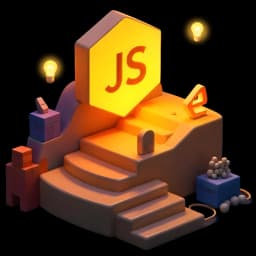
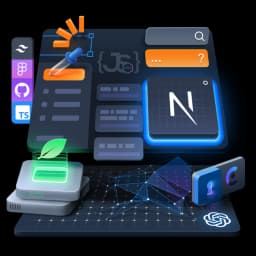
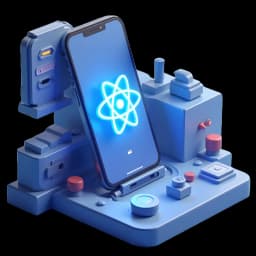
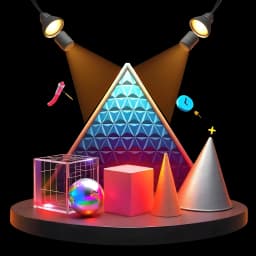
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access