Lifetime access to this course
Position
Let’s kick off our deep dive into transformations with one of the most essential — Position. In 3D space, nothing feels real until it’s been placed. You can’t just throw a cube into your scene and hope it ends up in the right spot. You have to tell it exactly where to go. That’s where positioning, or translation, comes in. --- ### 🗺️ What is Position ?
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
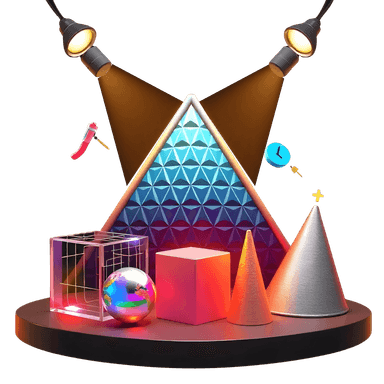
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
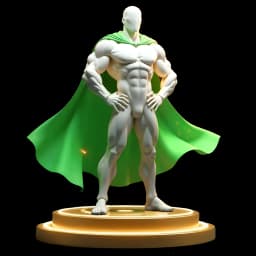
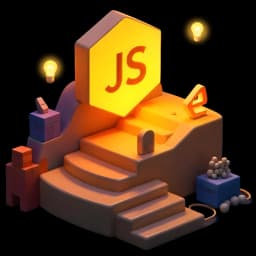
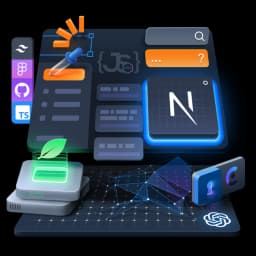
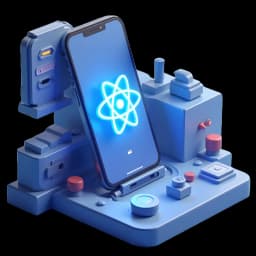
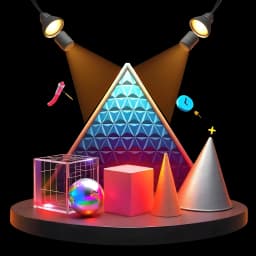
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access