Lifetime access to this course
Rotation
Welcome back! Now that you’ve got the hang of positioning objects in 3D space, let’s take it one step further — by learning how to rotate them. In this lesson, we’re diving into Rotation — one of the most powerful and visually exciting transformations in 3D graphics. Rotation allows you to spin, tilt, and turn objects in your scene, making everything feel more dynamic and alive. ---
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
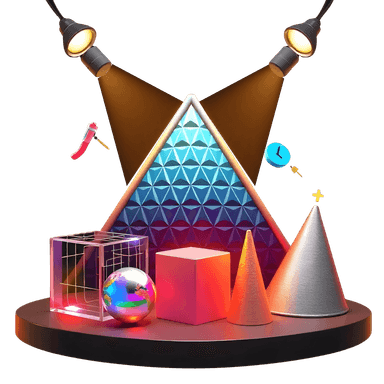
Vanilla Three.js Course
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
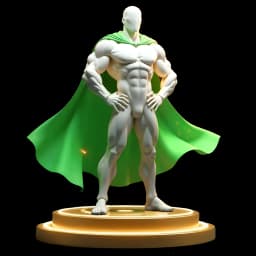
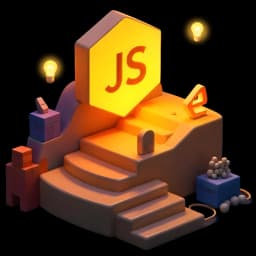
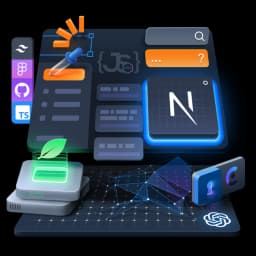
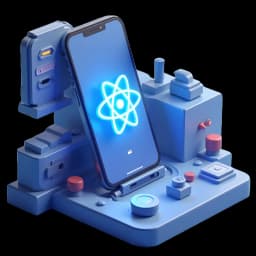
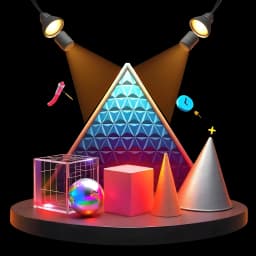
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access