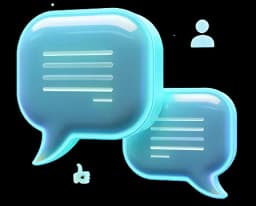
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Alright — we’ve moved things, we’ve rotated things…
Now it’s time to resize them.
Ever wanted to make a giant cube tower over your scene? Or shrink something down to the size of a marble? That’s what scaling is for — and it’s way easier than you might think.
Scaling is all about resizing objects — either uniformly (all sides equally), or non-uniformly (stretching/squishing in one direction).
In Three.js, every object has a .scale property — and it’s actually a THREE.Vector3. That means we can control the size on three axes:
So when we say:
cube.scale.set(2, 1, 1)
We’re saying: 👉 “Make it twice as wide, but keep the height and depth the same.”
Let’s stretch our cube a little:
cube.scale.x = 2; // Wide
cube.scale.y = 0.5; // Short
cube.scale.z = 1; // Normal depth
You can also scale all three axes at once using the .set() method:
cube.scale.set(2, 0.5, 1);
Quick, clean, and readable.
What if you want to make the whole object bigger or smaller without distorting its shape?
Just give all three axes the same number.
cube.scale.set(2, 2, 2); // Double size
cube.scale.set(0.5, 0.5, 0.5); // Half size
It’s perfect when you want things to stay proportional — like making a whole building model larger without turning it into a weird skyscraper-pancake hybrid.
Want to scale something based on how big it already is?
Use .multiplyScalar() — it multiplies the current scale by a value.
cube.scale.multiplyScalar(1.5); // Grows 50%
It’s like saying, “take whatever size it is now, and scale that.”
Scaling isn’t something you only use by itself.
You can stretch, move, and rotate an object — all at once — to create more dramatic effects. Let’s try it out:
cube.scale.set(2, 0.5, 1); // Wide & short
cube.position.set(0, -2, 0); // Lower it a bit
function animate() {
requestAnimationFrame(animate);
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
Notice how the stretched shape spins with a different vibe? Combining transformations like this is where 3D really comes alive.
Before you run off and scale everything like crazy — here are a few things to keep in mind:
Here’s a little challenge for you — a mix of scaling and animation:
Give it a shot. You’ll learn best by doing — and it’s fun to see the results come to life.
is a powerful tool in Three.js that allows you to resize and reshape objects in your 3D scenes. By mastering the property and understanding how works in 3D space, you’ll be able to create dynamic and engaging visuals.
In the next lesson, we’ll explore . Stay tuned, and happy coding!
See you there! 👋
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.