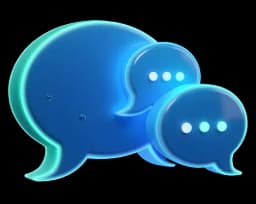
No Comments Yet
Be the first to share your thoughts and start the conversation.
Vapi has updated how Workflows are used. Be sure to review the latest implementation below!
export const generator = {
name: "Generate Interview",
nodes: [
{
name: "start",
type: "conversation",
isStart: true,
metadata: {
position: {
x: 0,
y: 0,
},
},
prompt:
"Speak first. Greet the user and help them create a new AI Interviewer",
voice: {
model: "aura-2",
voiceId: "thalia",
provider: "deepgram",
},
variableExtractionPlan: {
output: [
{
title: "level",
description: "The job experience level.",
type: "string",
enum: ["entry", "mid", "senior"],
},
{
title: "amount",
description: "How many questions would you like to generate?",
type: "number",
enum: [],
},
{
title: "techstack",
description:
"A list of technologies to cover during the job interview. For example, React, Next.js, Express.js, Node and so on...",
type: "string",
enum: [],
},
{
title: "role",
description:
"What role should would you like to train for? For example Frontend, Backend, Fullstack, Design, UX?",
type: "string",
enum: [],
},
{
title: "type",
description: "What type of the interview should it be? ",
type: "string",
enum: ["behavioural", "technical", "mixed"],
},
],
},
},
{
name: "apiRequest_1747470739045",
type: "apiRequest",
metadata: {
position: {
x: -16.075937072883846,
y: 703.623428447121,
},
},
method: "POST",
url: `${process.env.NEXT_PUBLIC_BASE_URL}/api/vapi/generate`,
headers: {
type: "object",
properties: {},
},
body: {
type: "object",
properties: {
role: {
type: "string",
description: "",
value: "{{ role }}",
},
level: {
type: "string",
description: "",
value: "{{ level }}",
},
type: {
type: "string",
description: "",
value: "{{ type }}",
},
amount: {
type: "number",
description: "",
value: "{{ amount }}",
},
userid: {
type: "string",
description: "",
value: "{{ userid }}",
},
techstack: {
type: "string",
description: "",
value: "{{ techstack }}",
},
},
},
output: {
type: "object",
properties: {},
},
mode: "blocking",
hooks: [],
},
{
name: "conversation_1747721261435",
type: "conversation",
metadata: {
position: {
x: -17.547788169718615,
y: 1003.3409337989506,
},
},
prompt:
"Thank the user for the conversation and inform them that the interview was generated successfully.",
voice: {
provider: "deepgram",
voiceId: "thalia",
model: "aura-2",
},
},
{
name: "conversation_1747744490967",
type: "conversation",
metadata: {
position: {
x: -11.165436030430953,
y: 484.94857971060617,
},
},
prompt: "Say that the Interview will be generated shortly.",
voice: {
provider: "deepgram",
voiceId: "thalia",
model: "aura-2",
},
},
{
name: "hangup_1747744730181",
type: "hangup",
metadata: {
position: {
x: 76.01267674000721,
y: 1272.0665127156606,
},
},
},
],
edges: [
{
from: "apiRequest_1747470739045",
to: "conversation_1747721261435",
condition: {
type: "ai",
prompt: "",
},
},
{
from: "start",
to: "conversation_1747744490967",
condition: {
type: "ai",
prompt: "If user provided all the required variables",
},
},
{
from: "conversation_1747744490967",
to: "apiRequest_1747470739045",
condition: {
type: "ai",
prompt: "",
},
},
{
from: "conversation_1747721261435",
to: "hangup_1747744730181",
condition: {
type: "ai",
prompt: "",
},
},
],
};
}
Be the first to share your thoughts and start the conversation.